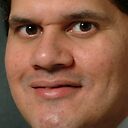
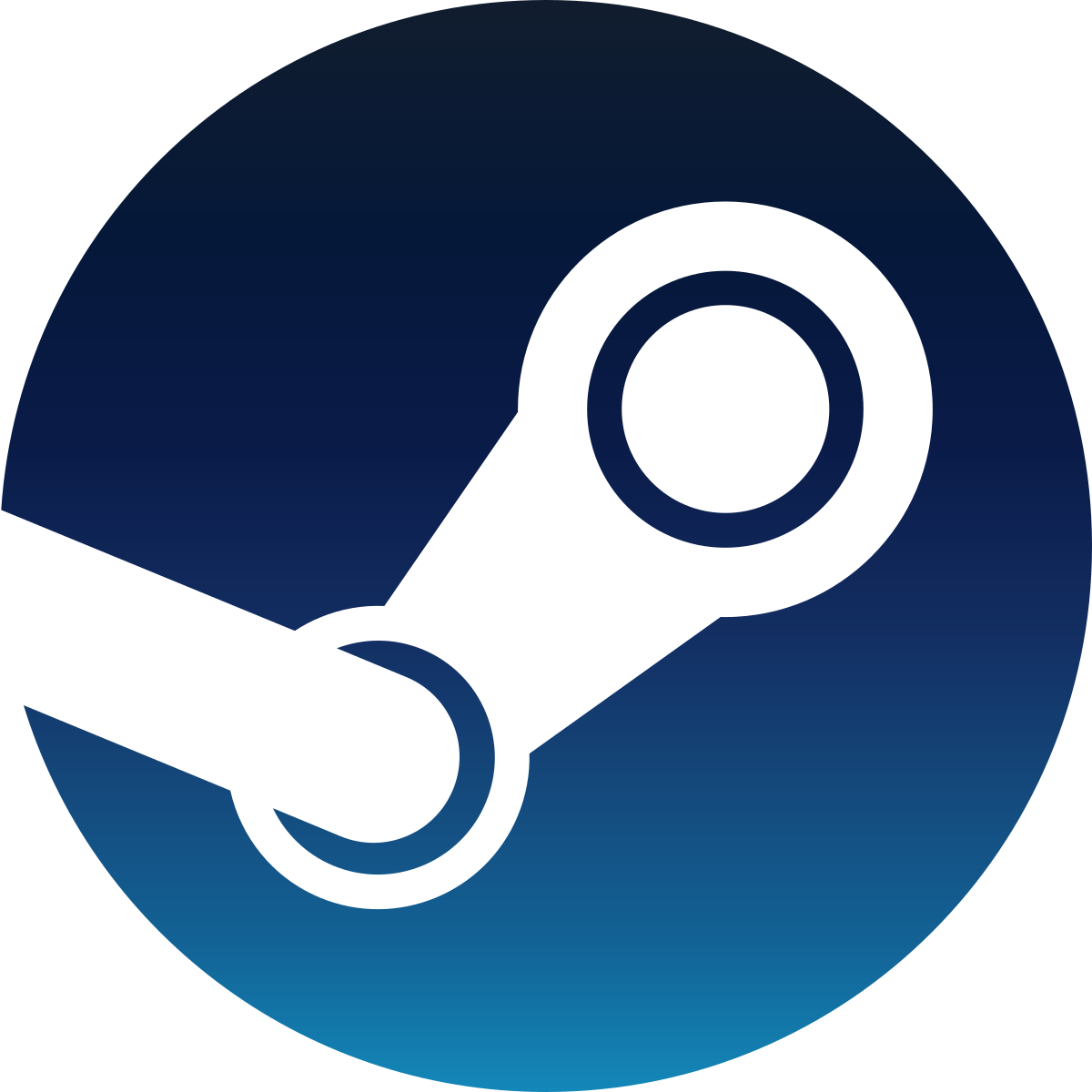
I want to avoid flatpacks, and I dont want to have to use it just for steam and nothing else.
I made the icy-nord and icy-nord-darker themes.
@promitheas:matrix.org
I want to avoid flatpacks, and I dont want to have to use it just for steam and nothing else.
This started happening to me 3 days ago. I did a full system update yesterday, as well as today, and still nothing different. I logged into the account from the browser normally, it immediately gave me the steam guard popup.
It does seem to be some kind of networking issue I think by looking at the output it spits out if I launch it from the terminal, but I just am not familiar with steam’s inner workings enough to know that for sure.
Whelp, turns out my government employees strike again! Turns out the whole registration is wrong. Its a 1993 but its registered as an EK3. No idea what might have happened there. Anyway, thanks for the info :D
Honestly Ive looked all over for my car manual but I cant find it anywhere on the internet. My car is a 1993 Honda Civic EK3 which I got second (more likely 5th or 10th) hand. The earliest model’s manual I can find online is the 1995 model. Do you know a good site that might have the owners manual for my model?
I thought it was the onion for a second there, but nope it’s t a real article
Is it weird that i dont want to be remembered? I just want to fade into nothingness. None of it matters anyway
This. Im not french, and while the surrender jokes can be slightly amusing at times, i feel a great sense of pride(?) everytime i see on the news that theyre protesting some new tyranny. Wish my lot were like that. Alas, we not only get trampled by the boot but kiss it as it passes by
Yea that seems like a good rule to have. Ill start doing that too, thanks
This was it. I got confused because I was using pacman -Q to search for the packages relating to qemu, but actually using yay to uninstall. Running simply pacman -R <package list> uninstalled them all without any dependency issues.
Could you please give me a short explanation of why that would happen when running it with an alias? Thanks!
Yes exactly! Thank you
How can you look through/search through all current initiatives without needing someone to supply a link?
That worked, thanks!
Im not sure about the launcher with built-in mod support as I dont plan on using any other mods, so Im not sure if its worth it for what is essentially a single mod and a shader pack.
Im on linux and the “Open folder” button doesnt work for each installation so that I can locate its files. However, if I “Edit” the Iris & Sodium instance I can see that the path for it is /home/mart/.minecraft
which is the same as for the initial Fabric instance. Is that not its installation directory? If not, how can I find the install directory, or where is it usually placed?
Here is what my /home/mart/.minecraft/ directory looks like:
My BetterF3 mod is in the mods
directory
Eve online for me too. Ill be logging in in a few minutes 😃
As for the social aspect, even though im in one the big alliances, if i just dont feel like it on any particular night then theres no need to be social. I can do my own thing.
I changed how i look at communities when i switched to lemmy. Instead of just looking at the part before the @ i look at the whole thing and consider that to be its name.
So instead of the gaming community its [email protected] and [email protected] as a whole.
Tl;dr: theyre different separate comminities and the name is for all intents and purposes the entire thing.
I suggest subscribing to all of them for the topics you want and slowly through exposure learning which ones youd like to keep.
Use chapstick
Read a book in public
Not go to gym
Play certain more “feminine” games
Those off the top of my head. I live in a nation of backwards idiots, so there for sure are more
Could someone compile a list of maintainers associated with israeli companies to highlight the hypocrisy of removing russian associated maintainers to comply with international sanctions, when the ICJ has an ongoing case of genocide against israel?
An increasingly assertive group of African nations are constraining the ability of Canadian companies to profit from resources
How could they dare to do such a thing?!? Isnt their only purpose to be a place for the good west to resourcep-rape? /s
The weird thing is it worked perfectly fine before. Discord crashed a d due to my setup (bspwm + sxhkd) it for some reason causes those to crash as well, so when i unlock i have no ability to shutdown properly, so i have to use the physical power button. When I turned it back on, poof steam didnt work. Probably an update that i did before the crash, which became active on reboot.